Change default schema markup in Ghost
This tutorial will teach you how to change the default schema markup in Ghost via code injection.
Introduction
Schema markup is code that helps search engine understand your website content. For example, adding the "Organization" schema data allows Google to display your organization information in search results, as shown in the image below:
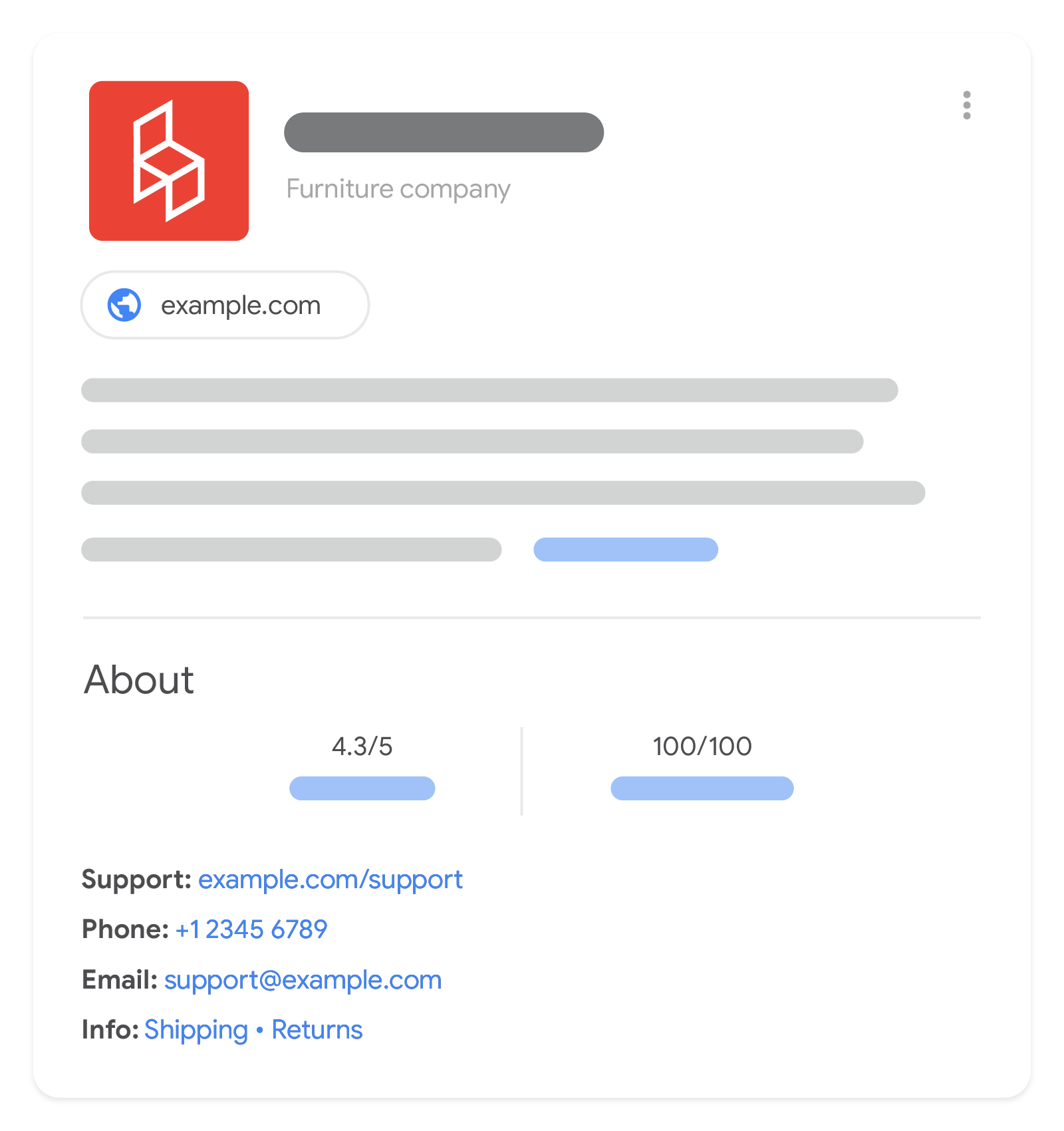
Ghost automatically generates schema markup using ghost_head, and you can view the generated schema markup by opening the "Developer Tools" in browsers and searching for "ld+json" under the "Elements" tab.
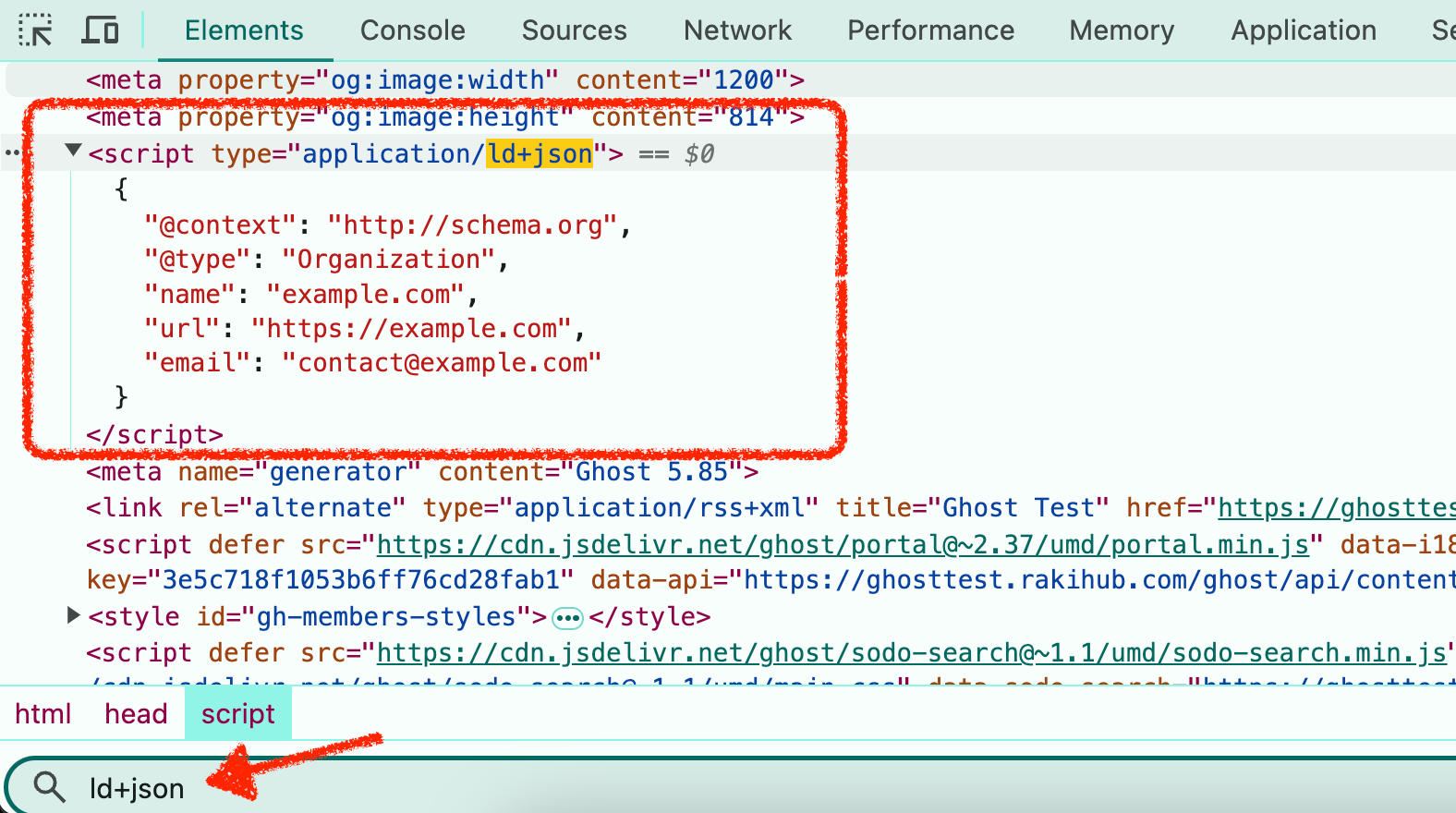
Change the default schema markup
Sometimes, you need to modify the default schema markup generated by Ghost. You can do this via code injection. For example, the default schema markup for https://source.ghost.io/ is like this:
<script type="application/ld+json">
{
"@context": "https://schema.org",
"@type": "WebSite",
"publisher": {
"@type": "Organization",
"name": "News Wave",
"url": "https://source.ghost.io/",
"logo": {
"@type": "ImageObject",
"url": "https://source.ghost.io/content/images/size/w256h256/2023/08/ghost-logo-orb.png",
"width": 60,
"height": 60
}
},
"url": "https://source.ghost.io/",
"image": {
"@type": "ImageObject",
"url": "https://static.ghost.org/v5.0.0/images/publication-cover.jpg",
"width": 1200,
"height": 840
},
"mainEntityOfPage": "https://source.ghost.io/",
"description": "Your trusted source for wide-ranging perspectives, thought-provoking analysis, and deep insights that resonate."
}
</script>
Replace 'publisher'
If you want to replace the whole "publisher" data inside the above code, you can inject the following code to Ghost settings --> Code injection --> Site footer.
<script>
// Select the script tag with type "application/ld+json"
const ldJsonScriptTag = document.querySelector('script[type="application/ld+json"]');
// Check if the tag was found
if (ldJsonScriptTag) {
// Parse the current content
let ldJsonContent = JSON.parse(ldJsonScriptTag.textContent);
// Update the publisher information
ldJsonContent.publisher = {
"@type": "Organization",
"name": "Updated Publisher",
"url": "https://updated.publisher.com/",
"email": "contact@example.com",
"logo": {
"@type": "ImageObject",
"url": "https://updated.publisher.com/logo.png",
"width": 60,
"height": 60
}
};
// Update the content of the script tag
ldJsonScriptTag.textContent = JSON.stringify(ldJsonContent, null, 2);
}
</script>
Modify or add data to "publisher"
If you want to change part of the "publisher" data or add new data to it, you can inject the following code to Ghost settings --> Code injection --> Site footer.
<script>
// Select the script tag with type "application/ld+json"
const ldJsonScriptTag = document.querySelector('script[type="application/ld+json"]');
// Check if the tag was found
if (ldJsonScriptTag) {
// Parse the current content
let ldJsonContent = JSON.parse(ldJsonScriptTag.textContent);
// Check if 'publisher' exist
if ('publisher' in ldJsonContent) {
// Update the publisher information
ldJsonContent.publisher.email = "contact@example.com";
ldJsonContent.publisher.logo = {
"@type": "ImageObject",
"url": "https://updated.publisher.com/logo.png",
"width": 60,
"height": 60
};
}
// Update the content of the script tag
ldJsonScriptTag.textContent = JSON.stringify(ldJsonContent, null, 2);
}
</script>
Test the schema markup
You can use Rich Result Test to check if Google detects your schema markup. If successful, you will an image like this:

Note: Another popular test tool is Schema Validator, but it can't detect modified schema markup (it can only detect the original schema markup) because it can't execute the injected script like Google does.